Welcome to the world of Python Programming Journey! Whether you’re new to coding or transitioning from another language, Python is a fantastic choice. Known for its simplicity, readability, and vast applications, it’s no wonder Python has become one of the most popular programming languages today. This beginner’s guide will help you embark on your Python journey with confidence through seven essential steps.
1. Why Choose Python?
Python stands out for its easy-to-understand syntax, making it ideal for beginners. It’s widely used in various fields such as web development, data analysis, artificial intelligence, and scientific computing. Its versatility and the supportive community around it mean you’re never alone in your learning journey.
2. Setting Up Your Environment: Python Programming Journey
To start coding in Python, you’ll need to set up your programming environment. This involves downloading and installing Python from the official website (python.org). For a beginner-friendly coding environment, consider installing an Integrated Development Environment (IDE) like PyCharm or VSCode, which provides helpful tools like syntax highlighting and code completion.
3. Writing Your First Python Program:
Your first program is traditionally a ‘Hello, World!’ script. It’s simple:

This line of code tells Python to display the text ‘Hello, World!’ on the screen. It’s a great way to familiarize yourself with the syntax and the process of running a Python script.
4. Understanding Python Basics:
In your journey to learn Python, grasping the basics is crucial. This section will break down key concepts including variables and data types, control structures, and functions.
- Variables and Data Types:
- Variables: In Python, a variable is like a container that holds data. You can store different types of information in these containers. For example,
name = "Alice"
assigns the string “Alice” to the variablename
. - Data Types: Python supports various data types. The most common ones include:
- Integers: Whole numbers, e.g.,
age = 30
. - Floats: Decimal numbers, e.g.,
height = 5.9
. - Strings: Textual data, e.g.,
greeting = "Hello, World!"
. - Lists: Ordered collections of items, e.g.,
colors = ["red", "green", "blue"]
. - Dictionaries: Key-value pairs, e.g.,
person = {"name": "Alice", "age": 30}
.
- Integers: Whole numbers, e.g.,
- Type Conversion: Sometimes, you may need to convert data from one type to another, like turning a string into an integer with
int("10")
.
- Variables: In Python, a variable is like a container that holds data. You can store different types of information in these containers. For example,
- Control Structures:
- If-Else Statements: These are used for decision-making in your code. For example:
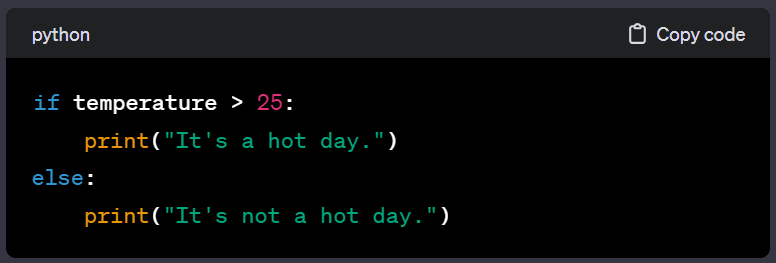
Loops: Loops are used for repeating a block of code multiple times.
- For Loops: Ideal for iterating over a sequence like a list.
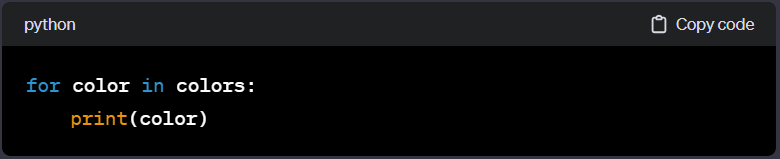
- While Loops: Continue executing as long as a condition is true.
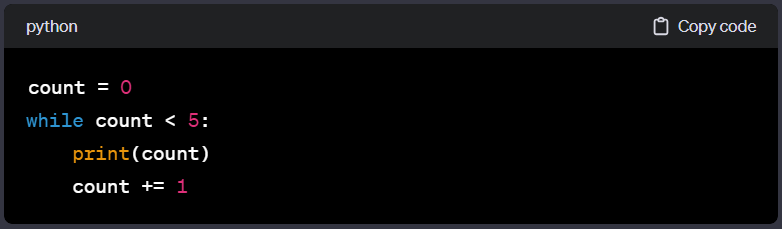
- Functions:
- Defining Functions: A function is a reusable block of code. You define a function using the
def
keyword, followed by a name, parentheses, and a colon. Inside the parentheses, you can pass arguments (variables) if needed.
- Defining Functions: A function is a reusable block of code. You define a function using the

- Calling Functions: To execute a function, you call it by its name and pass any required arguments.

- Return Values: Functions can return values using the
return
statement. For example, a function to add two numbers:

- You can then use this function and store its result:
sum = add(5, 3)
.
Understanding these basics forms the foundation of your Python programming skills. As you practice and experiment with these concepts, you’ll find yourself becoming more confident and capable in writing Python code.
5. Exploring Python Libraries:
One of Python’s strengths is its extensive libraries. Start with standard libraries like math
for mathematical operations and datetime
for dealing with dates and times. As you progress, explore powerful third-party libraries like NumPy for numerical computations and Pandas for data analysis.
6. Practical Projects:
The best way to learn is by doing. Start with simple projects like a calculator or a to-do list application. As you gain confidence, tackle more complex projects. Remember, each project enhances your understanding and problem-solving skills.
7. Joining the Python Community:
Engaging with the Python community can significantly enhance your learning. Join forums like Stack Overflow, participate in Python meetups, or contribute to open-source Python projects. The Python community is known for being welcoming and helpful.
Conclusion:
Starting with Python is an exciting journey. With its easy-to-learn syntax and powerful capabilities, you’re stepping into a world of endless possibilities. Remember, every expert was once a beginner, so be patient with yourself and enjoy the process of learning.